
Table of Contents
Navigation is an integral part of any mobile application, providing a pathway for users to traverse various screens and interfaces. React Navigation, a popular choice among developers, offers a flexible and intuitive approach to implementing complex navigation patterns with relative ease.
Building a Gatsby Blog Series
Laying the Foundation for React Navigation
Let's start by installing React Navigation:
npm install @react-navigation/native
For the Expo managed project, you will need to install additional dependencies:
expo install react-native-gesture-handler react-native-reanimated react-native-screens react-native-safe-area-context @react-native-community/masked-view
Grasping the Core of Navigation
Demystifying navigation elements: stacks, tabs, and drawers
These are the three primary navigation patterns in mobile applications:
- Stack Navigation: It follows a stack data structure where screens are stacked on top of each other. Navigating to a new screen places it on top of the stack, and you can go back by popping the screen off the stack.
- Tab Navigation: It is commonly used for the app's main navigation where different sections of the app are accessible via tabs typically located at the bottom of the screen.
- Drawer Navigation: It provides a sidebar drawer from the left or right of the screen, typically containing links to various sections of the app.
A primer on screens and components in the context of navigation
In React Navigation, a screen corresponds to a React component. It gets displayed when that screen's route is active in the navigation stack.
import * as React from 'react';import { Text, View } from 'react-native';
function HomeScreen() { return ( <View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}> <Text>Home Screen</Text> </View> );}
A peek into navigation options and parameters
We can pass parameters to routes using the navigate function.
navigation.navigate('RouteName', { /* parameters goes here */});
We can also customize the appearance of a screen in the stack using navigation options.
FAQ
- Why use React Navigation instead of other navigation libraries?
React Navigation provides a JavaScript-based navigation solution that doesn't need native code recompilation, making it a flexible and popular choice.
- Can I use both Tab and Stack navigation in the same app?
Absolutely. You can nest different navigation patterns within each other to achieve the desired navigation flow.
- How can I pass parameters to a screen?
Parameters can be passed as a second argument to the navigation.navigate
function, which will be available in the screen component's props.
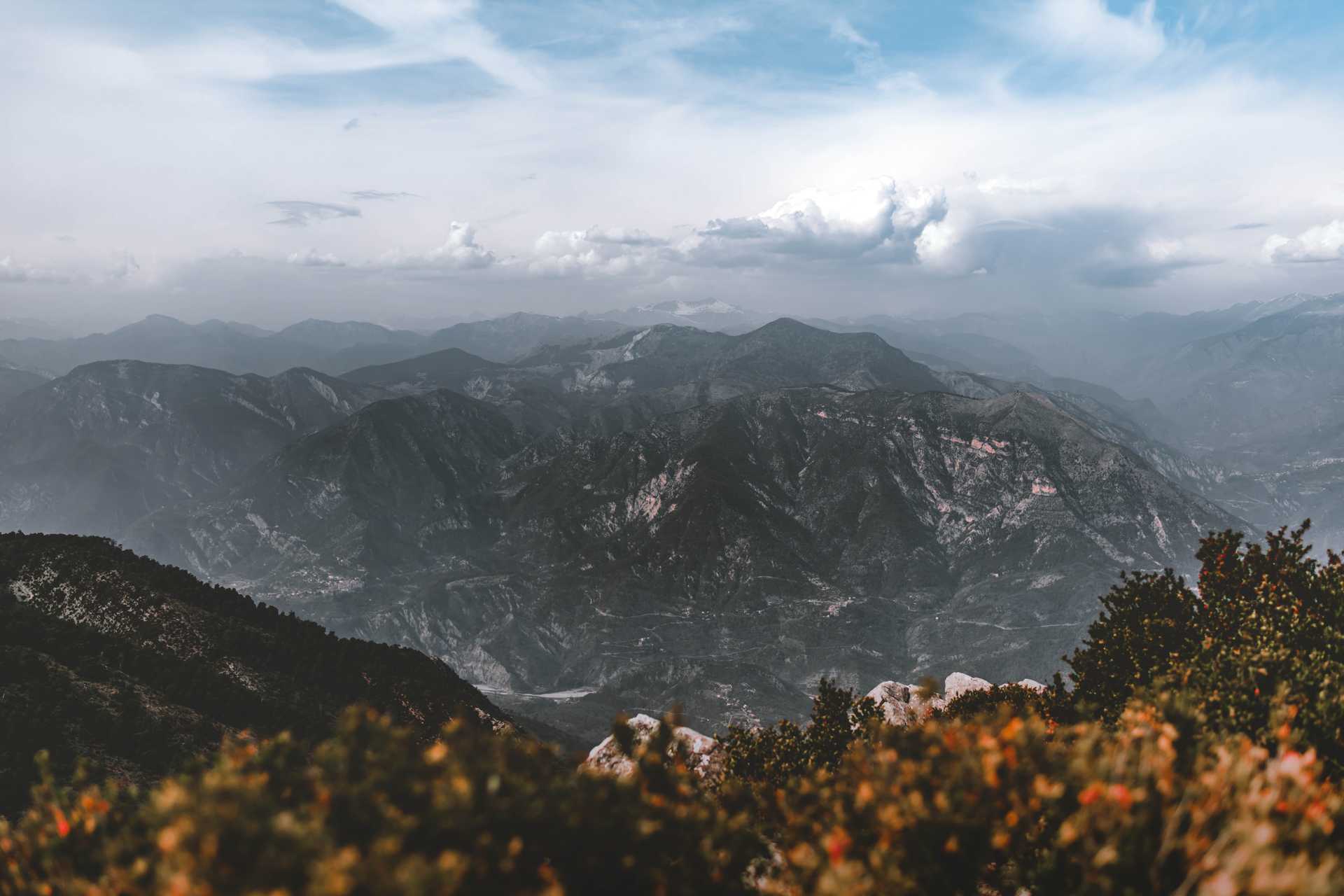

Web application development is a rapidly growing field that offers endless possibilities for creativity and innovation. From online shopping and banking to…